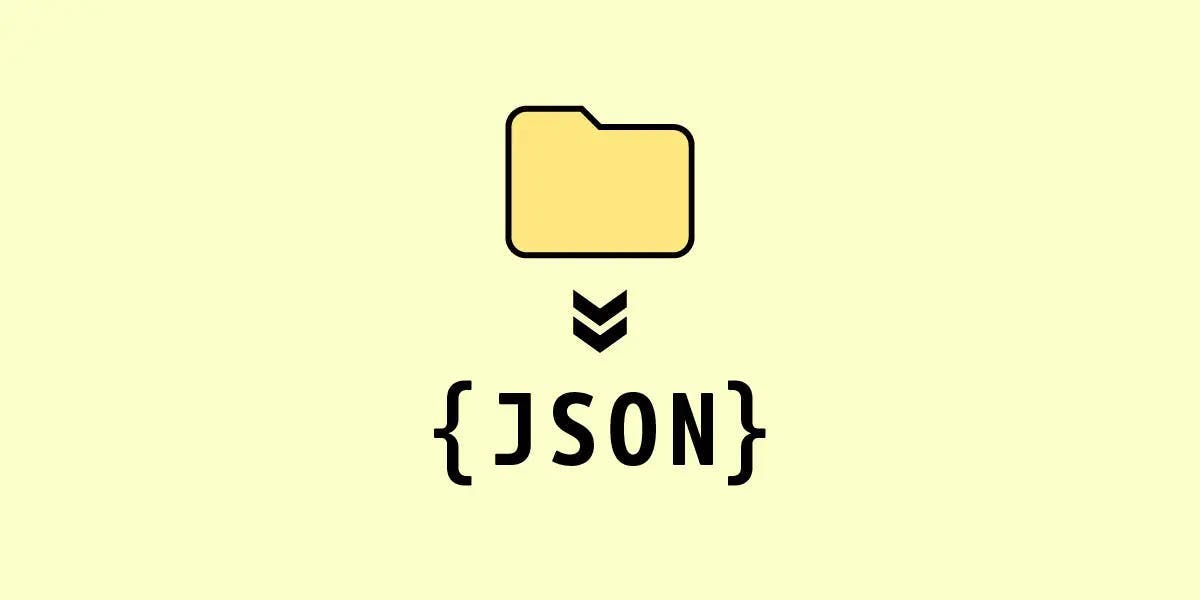
dir-to-json
dir-to-json
NPM package for asynchronously converting a directory tree structure into a JavaScript object.
Published / By Travis Wimer
Last updated
dir-to-json is an NPM package for asynchronously converting a directory tree structure into a JavaScript object.
I first published this package back in 2014 and I recently updated it to add TypeScript support and cleaner code.
It's a simple API:
import dirToJson from "dir-to-json";
async function run() {
const dirTree = await dirToJson("./path/to/my/dir");
console.log(JSON.stringify(dirTree, null, "\t"));
}
run();
This would be expected to log something like this:
{
"parent": "..",
"path": "",
"name": "coverage",
"type": "directory",
"children": [{
"parent": "",
"path": "coverage-final.json",
"name": "coverage-final.json",
"type": "file"
}, {
"parent": "",
"path": "index.html",
"name": "index.html",
"type": "file"
}, {
"parent": "",
"path": "lcov-report",
"name": "lcov-report",
"type": "directory",
"children": [{
"parent": "lcov-report",
"path": "lcov-report/index.html",
"name": "index.html",
"type": "file"
}, {
"parent": "lcov-report",
"path": "lcov-report/prettify.css",
"name": "prettify.css",
"type": "file"
}, {
"parent": "lcov-report",
"path": "lcov-report/prettify.js",
"name": "prettify.js",
"type": "file"
}, {
"parent": "lcov-report",
"path": "lcov-report/src",
"name": "src",
"type": "directory",
"children": [{
"parent": "lcov-report/src",
"path": "lcov-report/src/createDirectoryObject.js.html",
"name": "createDirectoryObject.js.html",
"type": "file"
}, {
"parent": "lcov-report/src",
"path": "lcov-report/src/index.html",
"name": "index.html",
"type": "file"
}, {
"parent": "lcov-report/src",
"path": "lcov-report/src/main.js.html",
"name": "main.js.html",
"type": "file"
}]
}]
}, {
"parent": "",
"path": "lcov.info",
"name": "lcov.info",
"type": "file"
}, {
"parent": "",
"path": "prettify.css",
"name": "prettify.css",
"type": "file"
}, {
"parent": "",
"path": "prettify.js",
"name": "prettify.js",
"type": "file"
}, {
"parent": "",
"path": "src",
"name": "src",
"type": "directory",
"children": [{
"parent": "src",
"path": "src/createDirectoryObject.js.html",
"name": "createDirectoryObject.js.html",
"type": "file"
}, {
"parent": "src",
"path": "src/index.html",
"name": "index.html",
"type": "file"
}, {
"parent": "src",
"path": "src/main.js.html",
"name": "main.js.html",
"type": "file"
}]
}]
}